Use an IR Receiver with Arduino
Receive controls from your remote control!
Written By: Cherie Tan
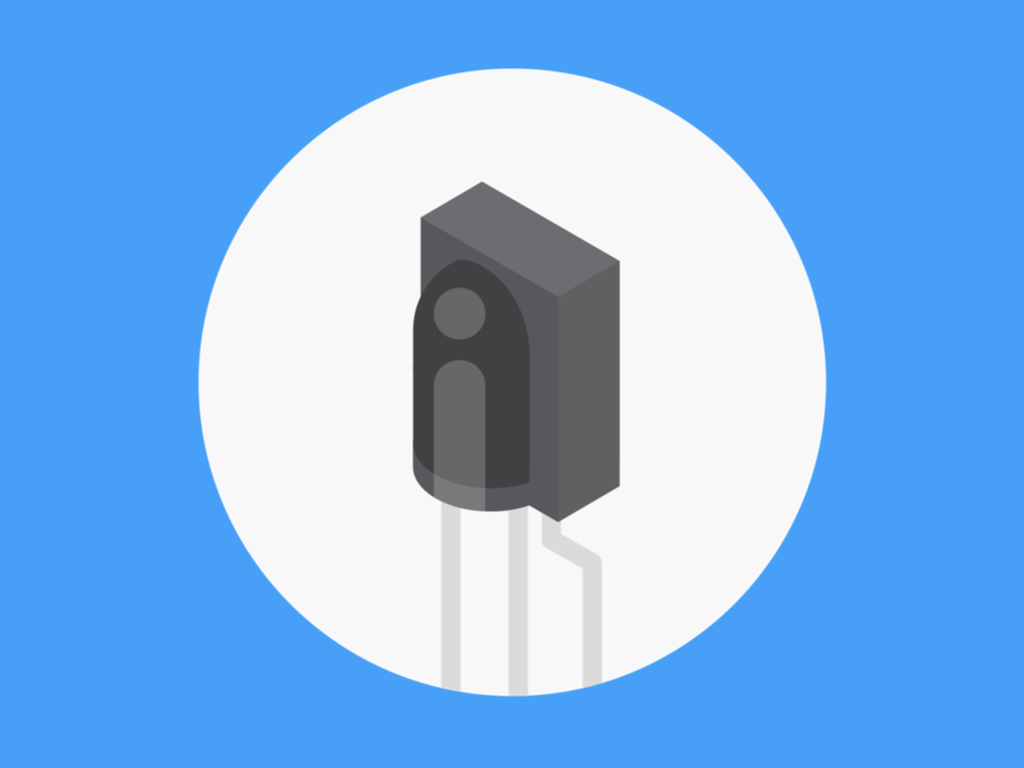

Difficulty
Easy

Steps
11
An IR receiver uses infrared communication. Infrared light, unlike visible light, has a slightly longer wavelength and so is undetectable to the human eye. But it is detectable by a TV which listens for IR signals.
In this guide, you will learn how to capture button presses on a remote with the 100% compatible Arduino development board, the Little Bird Uno R3 and an Arduino compatible IR receiver module. Have a try with a few different remote controls you have around the house!
After completing this guide, you will understand the basics involved and can use an IR receiver in your very own Arduino projects!
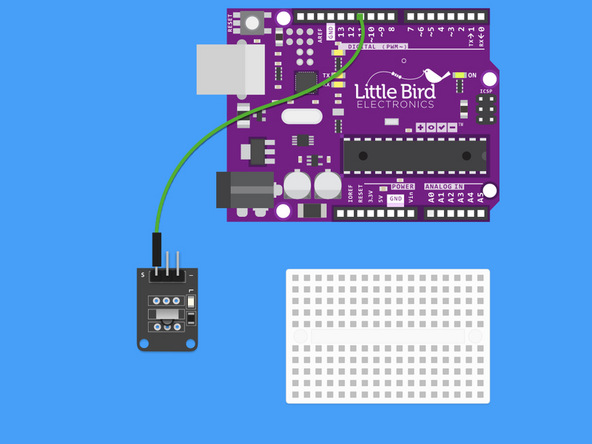
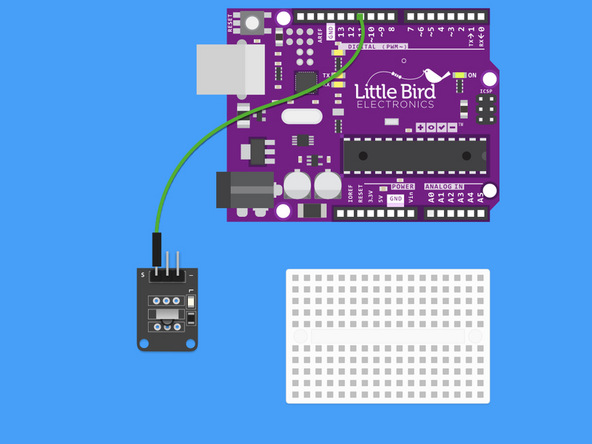
Connect Digital Pin 11 to the Signal Pin of the IR Receiver.
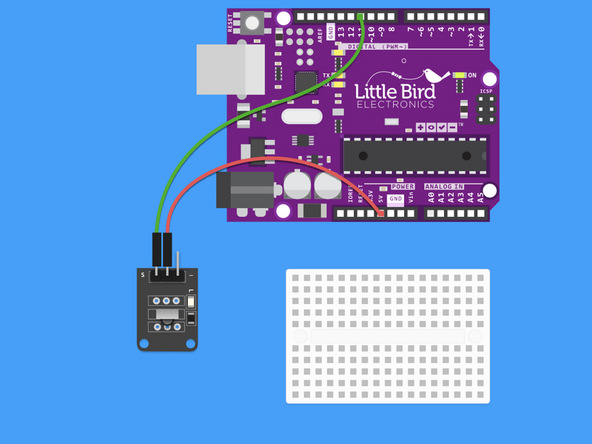
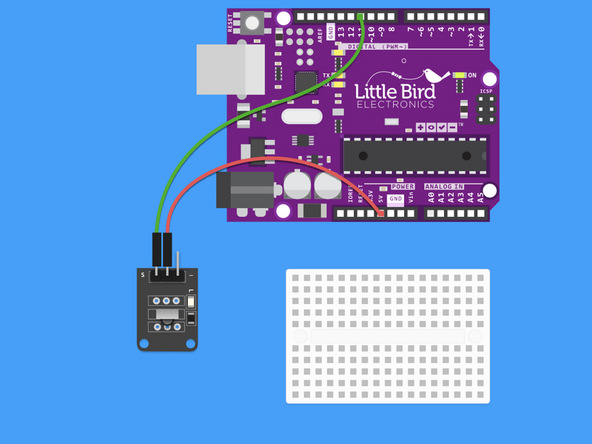
Connect 5V to the 5V (middle) Pin of the IR Receiver.
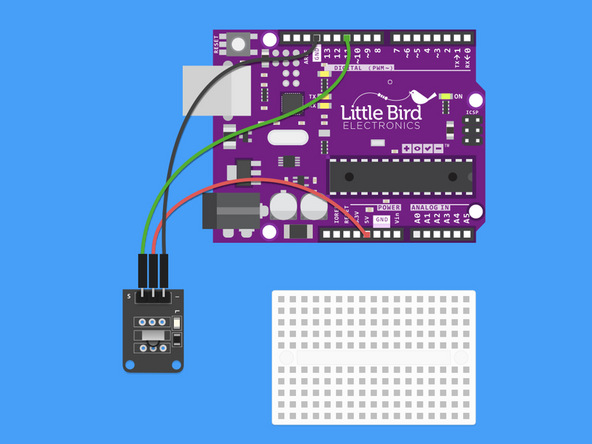
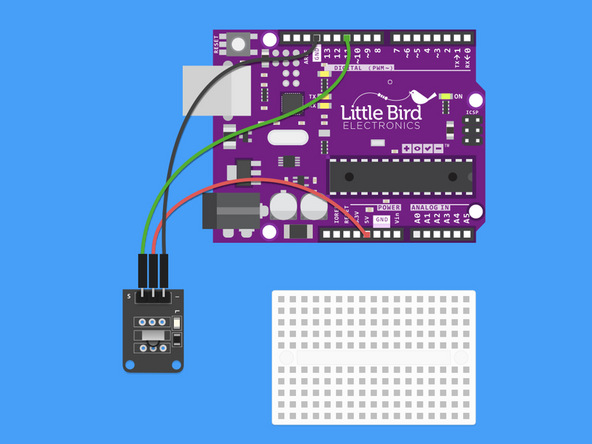
Connect Ground to the Ground Pin of the IR Receiver.
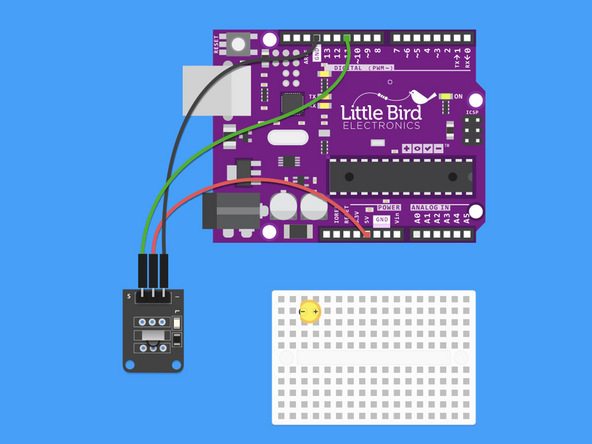
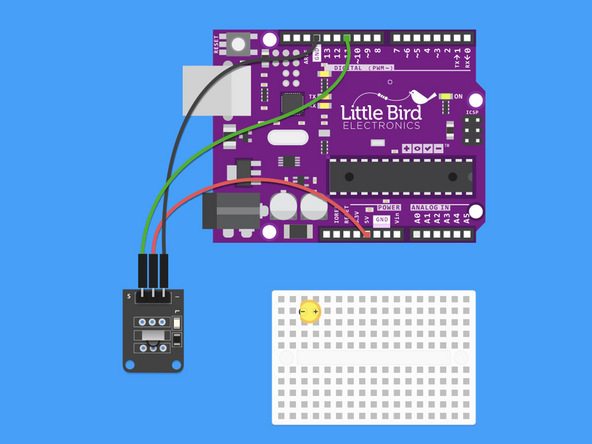
Insert the LED into the breadboard with the Cathode (shorter leg) on the left hand side.
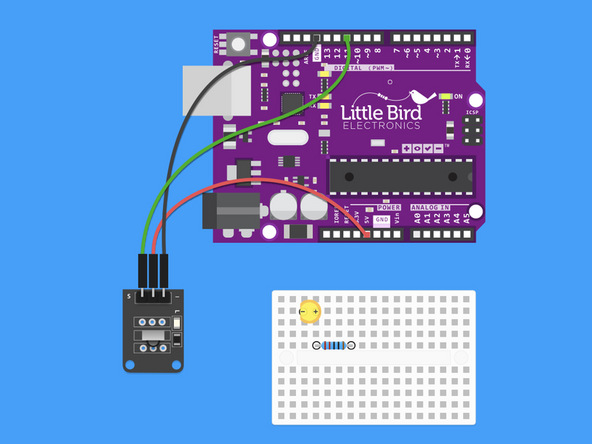
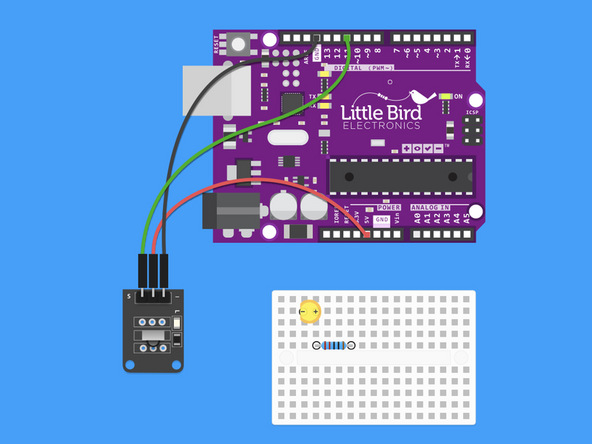
Insert a 220 Ohm Resistor into the Breadboard with one leg in line with the LED's anode (longer leg).
#include <IRremoteInt.h> #include <IRremote.h> int RECV_PIN = 11; //define input pin on Arduino IRrecv irrecv(RECV_PIN); decode_results results; void setup() { Serial.begin(9600); irrecv.enableIRIn(); // Start the receiver pinMode(LED_BUILTIN, OUTPUT); } void loop() { if (irrecv.decode( & results)) { String hex = String(results.value, HEX); Serial.print("Hexadecimal Code: "); Serial.println(hex); if(hex == "ff18e7"){ digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level) } if(hex == "ff4ab5"){ digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW } irrecv.resume(); // Receive the next value } }
Copy this code and open it in your Arduino IDE.
This code won't work without the IRremote Library (we'll install that next).
Open the Arduino Library Manager by clicking on Sketch → Include Libraries → Manage Libraries.
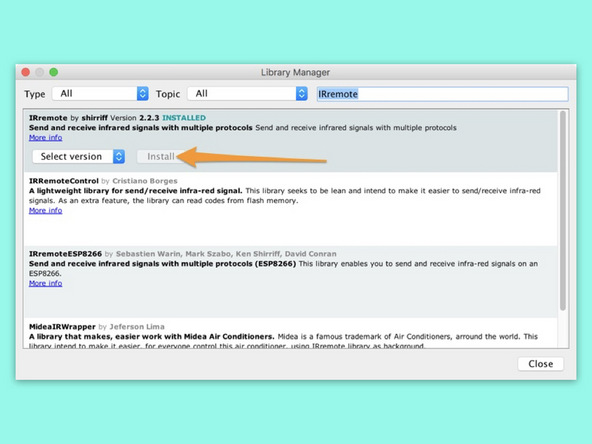
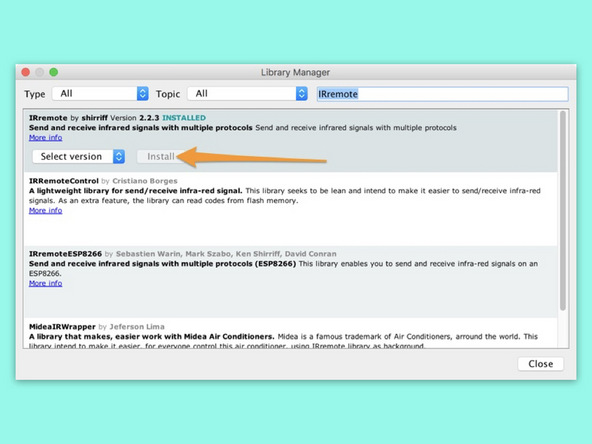
Search for the IRremote Library and install the latest version.